3.7 Hyperparameter Tuning
Contents
3.7 Hyperparameter Tuning#
In classic machine learning, models often have parameters that are learned from the training data (such as weights in a linear regression model), and hyperparameters that are external configuration settings. Hyperparameters are not learned from the data but are set prior to the training process and can significantly impact the model’s performance.
The goal of hyperparameter tuning is to find the optimal combination of hyperparameter values that maximizes the model’s performance on a given dataset. This process helps fine-tune the model to achieve the best possible results and avoid overfitting or underfitting.
Hyper-parameter tuning is now standard and should be performed in every work.
Several approaches exists to hyper-parameter tuning:
Manual Tuning:
While more time-consuming, manual tuning involves domain experts iteratively adjusting hyperparameters based on their understanding of the problem and the model’s behavior.
This approach can be effective when the hyperparameter space is relatively small or when there is substantial domain knowledge.
This is a good step for initial exploration and intuition-building experiment, but systematic approaches like described below will be required for robust assessment.
Grid Search:
In this method, a predefined set of hyperparameter values is specified, and the model is trained and evaluated for all possible combinations.
While thorough, grid search can be computationally expensive, especially for a large number of hyperparameters or when the search space is extensive.
This method is implemented in the
scikit-learn
ecosystem asmodel_selection.GridSearchCV
, which uses cross-validation to train-test each model given the tested hyper-parameters.
Random Search:
Random search involves randomly selecting combinations of hyperparameter values from a predefined distribution of hyperparameters (e.g., uniform or normal).
This approach is more computationally efficient than grid search, as it explores a diverse set of hyperparameter combinations.
The method is implement in the
scikit-learn
ecosystem asmodel_selection.RandomizedSearchCV
, which uses cross-validation for each
Bayesian Optimization:
Bayesian optimization employs probabilistic models to predict the performance of different hyperparameter configurations.
It adapts its search based on the results of previous evaluations, allowing it to focus on promising regions of the hyperparameter space.
Below is a tutorial using the
digits
data sets.
# basic tools
import numpy as np
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
# dataseta
from sklearn.datasets import load_digits
digits = load_digits()
digits.keys()
dict_keys(['data', 'target', 'frame', 'feature_names', 'target_names', 'images', 'DESCR'])
# explore data type
data,y = digits["data"].copy(),digits["target"].copy()
print(type(data[0][:]),type(y[0]))
# note that we do not modify the raw data that is stored on the digits dictionary.
<class 'numpy.ndarray'> <class 'numpy.int64'>
Plot the data
plt.matshow(digits["images"][1])
<matplotlib.image.AxesImage at 0x145352250>
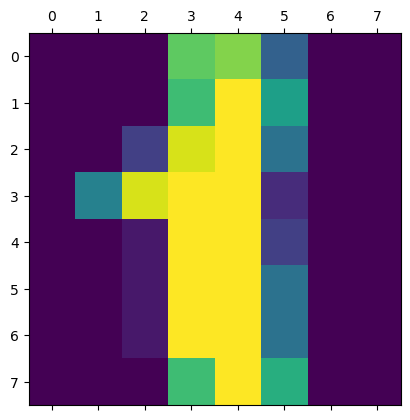
print(min(data[0]),max(data[0]))
from sklearn.preprocessing import MinMaxScaler
from sklearn.model_selection import train_test_split
scaler = MinMaxScaler()
scaler.fit_transform(data)# fit the model for data normalization
newdata = scaler.transform(data) # transform the data. watch that data was converted to a numpy array
# Split data into 50% train and 50% test subsets
print(f"There are {data.shape[0]} data samples")
X_train, X_test, y_train, y_test = train_test_split(
data, y, test_size=0.2, shuffle=False)
0.0 15.0
There are 1797 data samples
from sklearn import metrics
from sklearn.neighbors import KNeighborsClassifier
# Support Vector Machine classifier
clf = KNeighborsClassifier(n_neighbors=3)
clf.fit(X_train, y_train) # learn
knn_prediction = clf.predict(X_test) # predict on test
print("SVC Accuracy:", metrics.accuracy_score(y_true=y_test ,y_pred=knn_prediction))
SVC Accuracy: 0.9666666666666667
what are the parameters we are trying to optimize?
clf.get_params()
{'algorithm': 'auto',
'leaf_size': 30,
'metric': 'minkowski',
'metric_params': None,
'n_jobs': None,
'n_neighbors': 3,
'p': 2,
'weights': 'uniform'}
A search consists of:
an estimator (regressor or classifier such as
KNN()
);a parameter space;
a method for searching or sampling candidates (grid search or random selection);
a cross-validation scheme; and
a loss function or a scoring metrics.
1. Grid Search cross validation.#
Performs the search in the brute-force way using cross-validation. One has to define the parameter space. The scikit-learn function is GridSearchCV
. More details here.
from sklearn.model_selection import GridSearchCV
param_grid = [
{'n_neighbors': [1,2,3,4,5,6,7,8,9,10], 'weights': ['uniform','distance'], 'algorithm': [ 'ball_tree', 'kd_tree'],
'metric':['euclidean','manhattan','chebyshev','minkowski']}
]
The algorithm will search for all combinations of parameters, which can be from the model algorithms or the choice of features.
search = GridSearchCV(clf, param_grid, cv=5,verbose=3)
search.fit(X_train, y_train) # learn
Fitting 5 folds for each of 160 candidates, totalling 800 fits
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.972 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.941 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.986 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.986 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.906 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.941 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.972 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.913 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.941 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.969 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.986 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.941 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.930 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.930 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.930 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.917 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.913 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.913 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.937 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.913 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.937 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.937 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.937 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.913 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.955 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.951 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.958 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.962 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.937 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.951 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.958 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.955 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.951 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.958 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.944 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.955 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.917 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.944 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.930 total time= 0.1s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.944 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.920 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.951 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.923 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.951 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.927 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.955 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.958 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.930 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.972 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.941 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=ball_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=uniform;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=1, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=2, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.972 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=3, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.941 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=4, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=5, weights=distance;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=7, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=8, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=9, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=euclidean, n_neighbors=10, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.986 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.986 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.955 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.941 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=2, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.972 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=3, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.913 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.941 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=uniform;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.969 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=4, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.986 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=5, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.941 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.930 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.969 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.930 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=7, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.910 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.930 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=uniform;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.917 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=8, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.917 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=uniform;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.917 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.934 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=9, weights=distance;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.906 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.937 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.962 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.913 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.937 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.969 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=manhattan, n_neighbors=10, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.941 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.941 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=uniform;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.941 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.941 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=1, weights=distance;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.941 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.934 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=uniform;, score=0.951 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.934 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=2, weights=distance;, score=0.955 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.937 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=uniform;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.951 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=3, weights=distance;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=uniform;, score=0.958 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=4, weights=distance;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.948 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=uniform;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.958 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.958 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=5, weights=distance;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.955 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.941 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=uniform;, score=0.962 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.955 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=6, weights=distance;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.951 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.937 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.941 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=uniform;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.958 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.948 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.927 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=uniform;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.955 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.937 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=8, weights=distance;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.951 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.920 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=uniform;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.962 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.923 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=9, weights=distance;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.941 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.951 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.913 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=uniform;, score=0.965 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.955 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.923 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=chebyshev, n_neighbors=10, weights=distance;, score=0.969 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=uniform;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=1, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.948 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.983 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.965 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=2, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.972 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=uniform;, score=0.990 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.948 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=3, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=uniform;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.941 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.990 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.976 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=4, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=5, weights=distance;, score=0.976 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.938 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.965 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.948 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.944 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.965 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=6, weights=distance;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.934 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.951 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=7, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.920 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.976 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.955 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=8, weights=distance;, score=0.986 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.944 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=uniform;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.931 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.972 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.951 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=9, weights=distance;, score=0.983 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.924 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.969 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=uniform;, score=0.979 total time= 0.0s
[CV 1/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.927 total time= 0.0s
[CV 2/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.969 total time= 0.0s
[CV 3/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.944 total time= 0.0s
[CV 4/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.958 total time= 0.0s
[CV 5/5] END algorithm=kd_tree, metric=minkowski, n_neighbors=10, weights=distance;, score=0.983 total time= 0.0s
GridSearchCV(cv=5, estimator=KNeighborsClassifier(n_neighbors=3), param_grid=[{'algorithm': ['ball_tree', 'kd_tree'], 'metric': ['euclidean', 'manhattan', 'chebyshev', 'minkowski'], 'n_neighbors': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 'weights': ['uniform', 'distance']}], verbose=3)In a Jupyter environment, please rerun this cell to show the HTML representation or trust the notebook.
On GitHub, the HTML representation is unable to render, please try loading this page with nbviewer.org.
GridSearchCV(cv=5, estimator=KNeighborsClassifier(n_neighbors=3), param_grid=[{'algorithm': ['ball_tree', 'kd_tree'], 'metric': ['euclidean', 'manhattan', 'chebyshev', 'minkowski'], 'n_neighbors': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 'weights': ['uniform', 'distance']}], verbose=3)
KNeighborsClassifier(n_neighbors=3)
KNeighborsClassifier(n_neighbors=3)
search.get_params()
{'cv': 5,
'error_score': nan,
'estimator__algorithm': 'auto',
'estimator__leaf_size': 30,
'estimator__metric': 'minkowski',
'estimator__metric_params': None,
'estimator__n_jobs': None,
'estimator__n_neighbors': 3,
'estimator__p': 2,
'estimator__weights': 'uniform',
'estimator': KNeighborsClassifier(n_neighbors=3),
'n_jobs': None,
'param_grid': [{'n_neighbors': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10],
'weights': ['uniform', 'distance'],
'algorithm': ['ball_tree', 'kd_tree'],
'metric': ['euclidean', 'manhattan', 'chebyshev', 'minkowski']}],
'pre_dispatch': '2*n_jobs',
'refit': True,
'return_train_score': False,
'scoring': None,
'verbose': 3}
search.cv_results_
{'mean_fit_time': array([0.00140777, 0.00114398, 0.00129838, 0.0011826 , 0.00129204,
0.00114655, 0.00126467, 0.00125222, 0.00126867, 0.00128045,
0.00125637, 0.00133281, 0.00118542, 0.0012094 , 0.00116515,
0.00115423, 0.00118184, 0.00118876, 0.00122461, 0.00118771,
0.00127654, 0.00118356, 0.00120368, 0.00111895, 0.0011518 ,
0.00119176, 0.00112505, 0.00120587, 0.00110974, 0.00117784,
0.0011219 , 0.00117283, 0.00113697, 0.00110488, 0.00116277,
0.001121 , 0.00116334, 0.00110974, 0.00115099, 0.00111418,
0.00137367, 0.00133452, 0.00137486, 0.00139213, 0.00138397,
0.0013628 , 0.00139284, 0.00134025, 0.00137854, 0.00140023,
0.00136886, 0.00139098, 0.00155859, 0.00141668, 0.00142055,
0.00144706, 0.00151439, 0.00139289, 0.00139799, 0.00144835,
0.00118885, 0.00134029, 0.00118823, 0.00122175, 0.001193 ,
0.00112891, 0.00116839, 0.00116444, 0.00124407, 0.00117974,
0.00123711, 0.00120039, 0.00125556, 0.00111265, 0.00114856,
0.00115576, 0.00114322, 0.00117087, 0.00112543, 0.00114336,
0.00100203, 0.00109363, 0.00103416, 0.00109315, 0.00107622,
0.00105019, 0.00107789, 0.00106707, 0.00100613, 0.00108681,
0.0010047 , 0.00105281, 0.00098772, 0.00103245, 0.00107718,
0.00104809, 0.00108762, 0.00105462, 0.00108638, 0.0010766 ,
0.001086 , 0.00104437, 0.00114417, 0.00103259, 0.00102372,
0.00098853, 0.00109024, 0.00101366, 0.00104914, 0.00100737,
0.00107832, 0.00102644, 0.00103965, 0.000986 , 0.00104094,
0.00097799, 0.00103521, 0.00099483, 0.00104656, 0.00098691,
0.0010725 , 0.00096354, 0.00100341, 0.00097928, 0.00100942,
0.000951 , 0.00106764, 0.00100331, 0.00108519, 0.00092988,
0.00099473, 0.00099373, 0.00095 , 0.0010726 , 0.00095572,
0.00106049, 0.00096431, 0.00106978, 0.00103917, 0.00107574,
0.00106878, 0.00100555, 0.00106201, 0.00100651, 0.00109105,
0.0010776 , 0.0011312 , 0.00105872, 0.0011241 , 0.00100646,
0.00109911, 0.00099893, 0.00114799, 0.00103245, 0.00110121,
0.00106554, 0.00110216, 0.00105281, 0.00101843, 0.00103521]),
'std_fit_time': array([1.71090116e-04, 3.00189348e-05, 1.24715790e-04, 2.55285629e-05,
9.49969726e-05, 2.44506361e-05, 1.66215858e-04, 7.41443296e-05,
8.41001253e-05, 9.25170509e-05, 1.00821080e-04, 1.61908328e-04,
3.01314302e-05, 7.62891172e-05, 3.93108787e-05, 3.28915345e-05,
1.68216093e-05, 7.35214245e-05, 5.72436206e-05, 2.21452746e-05,
1.83254010e-04, 2.77388106e-05, 7.96841261e-05, 1.73789973e-05,
8.32625132e-05, 1.24529984e-04, 3.15201264e-05, 1.50838706e-04,
6.51122842e-06, 1.24211860e-04, 2.62618254e-05, 1.09887886e-04,
1.78724266e-05, 8.32268686e-06, 6.55140244e-05, 2.15123857e-05,
7.59943803e-05, 1.08846372e-05, 7.35851976e-05, 7.53553593e-06,
6.33518319e-05, 2.59758546e-05, 7.47469585e-05, 9.24755566e-05,
6.32655998e-05, 4.32410070e-05, 7.27390039e-05, 1.85382366e-05,
8.64354655e-05, 1.05876856e-04, 5.18281851e-05, 3.93043423e-05,
1.18209788e-04, 6.37087279e-05, 8.00219419e-05, 1.05915033e-04,
1.30014826e-04, 3.00643465e-05, 6.34298459e-05, 8.79932403e-05,
3.73823302e-06, 1.77316345e-04, 4.71659203e-06, 7.91938238e-05,
5.72028530e-05, 2.44576096e-05, 6.91363892e-05, 2.29363134e-05,
1.06741252e-04, 2.39486211e-05, 1.13813089e-04, 3.03447569e-05,
7.67524768e-05, 2.27408835e-05, 2.82940078e-05, 7.86269050e-05,
3.02883570e-05, 4.88405566e-05, 9.59924246e-06, 5.54700385e-05,
3.98629590e-05, 1.12701549e-04, 4.22501940e-05, 8.89347960e-05,
3.50087464e-05, 6.06278148e-05, 5.48530636e-05, 9.07951792e-05,
3.00153746e-05, 1.47165930e-04, 3.14593293e-05, 9.06615794e-05,
1.01795520e-05, 6.35863021e-05, 6.13200349e-05, 3.40953573e-05,
8.31993257e-05, 3.91336627e-05, 6.00077540e-05, 1.89950044e-05,
7.38942222e-05, 1.56331337e-05, 8.13463788e-05, 2.86149975e-05,
8.33335384e-05, 2.42674044e-05, 9.84424972e-05, 1.05213662e-05,
7.72581564e-05, 1.28581684e-05, 7.08186501e-05, 2.40575570e-05,
7.81141063e-05, 2.18126811e-05, 9.19044712e-05, 1.81304750e-05,
7.65587265e-05, 2.20294639e-05, 7.43035011e-05, 4.04372530e-05,
1.44617376e-04, 2.01660968e-05, 5.38320642e-05, 4.23810334e-05,
9.01360224e-05, 1.54501126e-05, 1.33724303e-04, 4.38346417e-05,
1.54175147e-04, 7.67752249e-06, 4.87549147e-05, 7.03978648e-05,
1.22247136e-05, 1.96723179e-04, 2.92820725e-05, 1.44483783e-04,
3.06078202e-05, 1.80232020e-04, 1.66980625e-04, 1.83111864e-04,
7.08200949e-05, 2.41369110e-05, 4.23439988e-05, 2.07561688e-05,
1.06092507e-04, 7.21619273e-05, 8.44819809e-05, 3.94268501e-05,
6.05826066e-05, 3.05658198e-05, 1.40552474e-04, 2.75116445e-05,
1.27419865e-04, 1.78909912e-05, 1.20704562e-04, 3.45216271e-05,
6.74254149e-05, 6.62246648e-05, 1.01630097e-05, 5.88218788e-05]),
'mean_score_time': array([0.0192246 , 0.01407104, 0.0192997 , 0.01457076, 0.01971579,
0.01445313, 0.02001534, 0.01503067, 0.01998377, 0.01503139,
0.02020388, 0.01521006, 0.02012463, 0.0151176 , 0.0198133 ,
0.0152606 , 0.02015944, 0.0153625 , 0.02068725, 0.01565228,
0.01888666, 0.0141058 , 0.01908689, 0.0142139 , 0.01911283,
0.01450577, 0.01936893, 0.01462278, 0.01936684, 0.01472216,
0.01948614, 0.01485596, 0.01973233, 0.01497216, 0.01983519,
0.01515818, 0.01998029, 0.01527653, 0.02011108, 0.01540737,
0.02948785, 0.0248394 , 0.02959356, 0.02505026, 0.03009968,
0.02515011, 0.02991104, 0.02514329, 0.02998571, 0.02530203,
0.03006983, 0.02562881, 0.03071399, 0.02577629, 0.0386919 ,
0.02588782, 0.030896 , 0.0258419 , 0.03060265, 0.02608404,
0.01905718, 0.01432905, 0.01929832, 0.01455708, 0.01921201,
0.01437521, 0.01928897, 0.01483183, 0.02005477, 0.01484685,
0.01985712, 0.01510458, 0.02002053, 0.01493359, 0.01975498,
0.01508956, 0.01980486, 0.01526103, 0.01991944, 0.0152317 ,
0.02172494, 0.0171936 , 0.02311587, 0.01831212, 0.02365112,
0.01843243, 0.02386303, 0.01875048, 0.02381821, 0.01887064,
0.02400956, 0.01897316, 0.02379055, 0.01928401, 0.02488422,
0.01961274, 0.02442665, 0.0198184 , 0.02464504, 0.01990356,
0.02304196, 0.018297 , 0.02335019, 0.018471 , 0.02313848,
0.01833758, 0.02333975, 0.01862588, 0.02344284, 0.01873102,
0.02366824, 0.01874394, 0.02355585, 0.01880918, 0.02355795,
0.01882615, 0.02369399, 0.01899896, 0.02380972, 0.01901288,
0.02041302, 0.01576381, 0.02243166, 0.01758518, 0.02328081,
0.01845818, 0.02421236, 0.0195549 , 0.02578182, 0.01971922,
0.02499743, 0.02032981, 0.02541871, 0.0211823 , 0.02574883,
0.02111402, 0.02635684, 0.02145925, 0.02673278, 0.02210412,
0.02214394, 0.01721282, 0.02275643, 0.01812615, 0.02359171,
0.02095075, 0.02392955, 0.01913462, 0.02415328, 0.01887522,
0.02377076, 0.01913071, 0.02424603, 0.01947122, 0.02480783,
0.0195775 , 0.02446957, 0.0196908 , 0.02453833, 0.01957202]),
'std_score_time': array([6.75307036e-04, 1.12750503e-04, 2.35445482e-04, 1.25485059e-04,
5.73434481e-04, 9.96180501e-05, 8.06490653e-04, 2.81525401e-05,
1.92780079e-04, 1.15094544e-04, 1.18115211e-04, 2.99947629e-05,
7.49559702e-04, 1.05411581e-04, 2.24663131e-04, 2.70611057e-04,
1.54478269e-04, 5.85430983e-05, 3.35284738e-04, 4.39610759e-05,
1.05888410e-04, 1.82295974e-04, 3.10639533e-04, 1.15908766e-04,
6.49099562e-05, 8.27834627e-05, 4.67131969e-04, 1.33900561e-04,
1.08064872e-04, 1.28512736e-04, 1.50243315e-04, 1.14831120e-04,
1.30773284e-04, 8.27937344e-05, 1.03093848e-04, 9.15293862e-05,
1.31771048e-04, 6.28460883e-05, 7.86090316e-05, 1.14788620e-04,
1.13003767e-04, 1.21687591e-04, 3.11070377e-05, 6.92233225e-05,
7.27641682e-04, 1.78425838e-04, 1.71644621e-04, 4.18068457e-05,
8.67049934e-05, 1.48252852e-04, 1.36335407e-04, 7.69596561e-05,
2.36095430e-04, 6.32968954e-05, 1.57373200e-02, 4.30767943e-05,
3.61636910e-04, 1.82123482e-04, 1.95001412e-04, 7.99469224e-05,
6.61107851e-05, 7.21113694e-05, 1.33004834e-04, 6.55957757e-05,
2.42195117e-04, 8.48094800e-05, 1.33071950e-04, 1.08310640e-04,
8.31048681e-04, 5.67392941e-05, 2.01330530e-04, 7.68001571e-05,
1.90305907e-04, 8.12745122e-05, 7.55171570e-05, 1.19239174e-04,
8.29365818e-05, 1.21450115e-04, 1.13455924e-04, 2.81909580e-05,
2.34400217e-04, 3.41992596e-04, 1.56933621e-04, 2.54237061e-04,
1.87265918e-04, 1.50998857e-04, 2.33459512e-04, 1.59470943e-04,
4.27112239e-04, 1.57037499e-04, 5.58690191e-04, 1.19450078e-04,
7.57933225e-05, 1.10427394e-04, 8.94337144e-04, 1.28664273e-04,
7.77653136e-05, 1.74009149e-04, 1.39781005e-04, 8.90700158e-05,
8.16783219e-05, 4.92495460e-05, 6.99964465e-05, 1.95514458e-04,
1.38236679e-04, 8.11215633e-05, 5.42113730e-05, 4.42250976e-05,
1.21882842e-04, 8.41197511e-05, 8.99606599e-05, 6.75788452e-05,
1.13176935e-04, 1.11128084e-04, 7.39198186e-05, 6.44952179e-05,
5.64075181e-05, 6.40352714e-05, 2.54606369e-04, 1.17968626e-04,
3.98965852e-04, 5.46154748e-04, 5.24937819e-04, 5.97699003e-04,
6.44012308e-04, 4.58953285e-04, 5.35265098e-04, 7.14581385e-04,
2.60857280e-03, 4.34593598e-04, 5.57278414e-04, 4.13535513e-04,
4.67071852e-04, 6.97260277e-04, 4.58880384e-04, 5.10349180e-04,
3.26138302e-04, 4.50128686e-04, 2.98156960e-04, 4.04460142e-04,
3.43651998e-04, 2.98076449e-04, 2.70522483e-04, 1.37570999e-04,
2.85702362e-04, 3.59473293e-03, 3.09399618e-04, 6.74778663e-05,
3.29866468e-04, 1.19612071e-04, 1.88306815e-04, 1.39061200e-04,
1.95032425e-04, 8.00878350e-05, 6.60324221e-04, 1.49858699e-04,
2.11504743e-04, 2.21621959e-04, 1.67262241e-04, 1.11046253e-04]),
'param_algorithm': masked_array(data=['ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'ball_tree', 'ball_tree', 'ball_tree', 'ball_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree',
'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree', 'kd_tree'],
mask=[False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False],
fill_value='?',
dtype=object),
'param_metric': masked_array(data=['euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'euclidean', 'euclidean', 'euclidean', 'euclidean',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'manhattan', 'manhattan', 'manhattan', 'manhattan',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'chebyshev', 'chebyshev', 'chebyshev', 'chebyshev',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski',
'minkowski', 'minkowski', 'minkowski', 'minkowski'],
mask=[False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False],
fill_value='?',
dtype=object),
'param_n_neighbors': masked_array(data=[1, 1, 2, 2, 3, 3, 4, 4, 5, 5, 6, 6, 7, 7, 8, 8, 9, 9,
10, 10, 1, 1, 2, 2, 3, 3, 4, 4, 5, 5, 6, 6, 7, 7, 8, 8,
9, 9, 10, 10, 1, 1, 2, 2, 3, 3, 4, 4, 5, 5, 6, 6, 7, 7,
8, 8, 9, 9, 10, 10, 1, 1, 2, 2, 3, 3, 4, 4, 5, 5, 6, 6,
7, 7, 8, 8, 9, 9, 10, 10, 1, 1, 2, 2, 3, 3, 4, 4, 5, 5,
6, 6, 7, 7, 8, 8, 9, 9, 10, 10, 1, 1, 2, 2, 3, 3, 4, 4,
5, 5, 6, 6, 7, 7, 8, 8, 9, 9, 10, 10, 1, 1, 2, 2, 3, 3,
4, 4, 5, 5, 6, 6, 7, 7, 8, 8, 9, 9, 10, 10, 1, 1, 2, 2,
3, 3, 4, 4, 5, 5, 6, 6, 7, 7, 8, 8, 9, 9, 10, 10],
mask=[False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False],
fill_value='?',
dtype=object),
'param_weights': masked_array(data=['uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance',
'uniform', 'distance', 'uniform', 'distance'],
mask=[False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False,
False, False, False, False, False, False, False, False],
fill_value='?',
dtype=object),
'params': [{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 10,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'manhattan',
'n_neighbors': 10,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'chebyshev',
'n_neighbors': 10,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'ball_tree',
'metric': 'minkowski',
'n_neighbors': 10,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'euclidean',
'n_neighbors': 10,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'manhattan',
'n_neighbors': 10,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'chebyshev',
'n_neighbors': 10,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 1,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 1,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 2,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 2,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 3,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 3,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 4,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 4,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 5,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 5,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 6,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 6,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 7,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 7,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 8,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 8,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 9,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 9,
'weights': 'distance'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 10,
'weights': 'uniform'},
{'algorithm': 'kd_tree',
'metric': 'minkowski',
'n_neighbors': 10,
'weights': 'distance'}],
'split0_test_score': array([0.94791667, 0.94791667, 0.9375 , 0.94791667, 0.94444444,
0.94444444, 0.93055556, 0.94097222, 0.9375 , 0.9375 ,
0.9375 , 0.94444444, 0.93402778, 0.93402778, 0.92013889,
0.93055556, 0.93055556, 0.93055556, 0.92361111, 0.92708333,
0.92361111, 0.92361111, 0.90625 , 0.92361111, 0.93402778,
0.93402778, 0.91319444, 0.92361111, 0.92013889, 0.92013889,
0.90972222, 0.92013889, 0.90972222, 0.90972222, 0.90972222,
0.91666667, 0.91319444, 0.91319444, 0.90972222, 0.91319444,
0.92361111, 0.92361111, 0.91319444, 0.93402778, 0.92013889,
0.93055556, 0.92708333, 0.92708333, 0.93055556, 0.93055556,
0.92708333, 0.92708333, 0.92361111, 0.92361111, 0.91666667,
0.92361111, 0.92708333, 0.92361111, 0.92013889, 0.92361111,
0.94791667, 0.94791667, 0.9375 , 0.94791667, 0.94444444,
0.94444444, 0.93055556, 0.94097222, 0.9375 , 0.9375 ,
0.9375 , 0.94444444, 0.93402778, 0.93402778, 0.92013889,
0.93055556, 0.93055556, 0.93055556, 0.92361111, 0.92708333,
0.94791667, 0.94791667, 0.9375 , 0.94791667, 0.94444444,
0.94444444, 0.93055556, 0.94097222, 0.9375 , 0.9375 ,
0.9375 , 0.94444444, 0.93402778, 0.93402778, 0.92013889,
0.93055556, 0.93055556, 0.93055556, 0.92361111, 0.92708333,
0.92361111, 0.92361111, 0.90972222, 0.92361111, 0.93402778,
0.93402778, 0.91319444, 0.92361111, 0.92361111, 0.92361111,
0.90972222, 0.92013889, 0.90972222, 0.90972222, 0.90972222,
0.91666667, 0.91666667, 0.91666667, 0.90625 , 0.91319444,
0.94097222, 0.94097222, 0.92361111, 0.93402778, 0.94444444,
0.95138889, 0.93055556, 0.93402778, 0.93402778, 0.93402778,
0.92361111, 0.92708333, 0.92708333, 0.92708333, 0.93402778,
0.9375 , 0.92361111, 0.93402778, 0.94097222, 0.9375 ,
0.94791667, 0.94791667, 0.9375 , 0.94791667, 0.94444444,
0.94444444, 0.93055556, 0.94097222, 0.9375 , 0.9375 ,
0.9375 , 0.94444444, 0.93402778, 0.93402778, 0.92013889,
0.93055556, 0.93055556, 0.93055556, 0.92361111, 0.92708333]),
'split1_test_score': array([0.98263889, 0.98263889, 0.98958333, 0.98263889, 0.98958333,
0.98958333, 0.97569444, 0.98958333, 0.97569444, 0.97916667,
0.96527778, 0.97916667, 0.96875 , 0.97222222, 0.96875 ,
0.97569444, 0.96875 , 0.97222222, 0.96875 , 0.96875 ,
0.98611111, 0.98611111, 0.97222222, 0.98958333, 0.98958333,
0.98958333, 0.97222222, 0.98958333, 0.97222222, 0.98611111,
0.96527778, 0.98263889, 0.96180556, 0.97222222, 0.96527778,
0.96875 , 0.96527778, 0.96875 , 0.96527778, 0.96527778,
0.96527778, 0.96527778, 0.95486111, 0.95833333, 0.96180556,
0.96527778, 0.96180556, 0.96180556, 0.95138889, 0.96527778,
0.96180556, 0.96180556, 0.94444444, 0.95486111, 0.94444444,
0.94444444, 0.94444444, 0.95138889, 0.95138889, 0.95833333,
0.98263889, 0.98263889, 0.98958333, 0.98263889, 0.98958333,
0.98958333, 0.97569444, 0.98958333, 0.97569444, 0.97916667,
0.96527778, 0.97916667, 0.96875 , 0.97222222, 0.96875 ,
0.97569444, 0.96875 , 0.97222222, 0.96875 , 0.96875 ,
0.98263889, 0.98263889, 0.98958333, 0.98263889, 0.98958333,
0.98958333, 0.97569444, 0.98958333, 0.97569444, 0.97916667,
0.96527778, 0.97916667, 0.96875 , 0.97222222, 0.96875 ,
0.97569444, 0.96875 , 0.97222222, 0.96875 , 0.96875 ,
0.98611111, 0.98611111, 0.97222222, 0.98958333, 0.98958333,
0.98958333, 0.97222222, 0.98958333, 0.97222222, 0.98611111,
0.96875 , 0.98263889, 0.96180556, 0.97222222, 0.96527778,
0.96875 , 0.96527778, 0.96875 , 0.96527778, 0.96527778,
0.96875 , 0.96875 , 0.96527778, 0.96527778, 0.96875 ,
0.96875 , 0.96527778, 0.96527778, 0.94791667, 0.95833333,
0.95486111, 0.95486111, 0.95138889, 0.95833333, 0.94791667,
0.95486111, 0.95138889, 0.96180556, 0.95138889, 0.95486111,
0.98263889, 0.98263889, 0.98958333, 0.98263889, 0.98958333,
0.98958333, 0.97569444, 0.98958333, 0.97569444, 0.97916667,
0.96527778, 0.97916667, 0.96875 , 0.97222222, 0.96875 ,
0.97569444, 0.96875 , 0.97222222, 0.96875 , 0.96875 ]),
'split2_test_score': array([0.9825784 , 0.9825784 , 0.96515679, 0.9825784 , 0.97212544,
0.97560976, 0.95121951, 0.97560976, 0.94425087, 0.96515679,
0.94773519, 0.96515679, 0.94425087, 0.95121951, 0.94425087,
0.94425087, 0.94425087, 0.94425087, 0.94425087, 0.94425087,
0.95818815, 0.95818815, 0.95818815, 0.95818815, 0.96515679,
0.97212544, 0.94076655, 0.96864111, 0.93379791, 0.94773519,
0.93379791, 0.94076655, 0.93031359, 0.93031359, 0.93031359,
0.93379791, 0.93379791, 0.93379791, 0.93728223, 0.93728223,
0.96515679, 0.96515679, 0.94425087, 0.96167247, 0.94773519,
0.95818815, 0.95818815, 0.95818815, 0.94773519, 0.95818815,
0.94425087, 0.95121951, 0.93379791, 0.94773519, 0.93031359,
0.93379791, 0.91986063, 0.92334495, 0.92682927, 0.93031359,
0.9825784 , 0.9825784 , 0.96515679, 0.9825784 , 0.97212544,
0.97560976, 0.95121951, 0.97560976, 0.94425087, 0.96515679,
0.94773519, 0.96515679, 0.94425087, 0.95121951, 0.94425087,
0.94425087, 0.94425087, 0.94425087, 0.94425087, 0.94425087,
0.9825784 , 0.9825784 , 0.96515679, 0.9825784 , 0.97212544,
0.97560976, 0.95121951, 0.97560976, 0.94425087, 0.96515679,
0.94773519, 0.96515679, 0.94425087, 0.95121951, 0.94425087,
0.94425087, 0.94425087, 0.94425087, 0.94425087, 0.94425087,
0.95818815, 0.95818815, 0.95470383, 0.95818815, 0.96515679,
0.97212544, 0.94076655, 0.96864111, 0.93379791, 0.94773519,
0.93379791, 0.94076655, 0.93031359, 0.93031359, 0.93031359,
0.93379791, 0.93379791, 0.93379791, 0.93728223, 0.93728223,
0.96515679, 0.96515679, 0.94076655, 0.95818815, 0.93728223,
0.94773519, 0.95818815, 0.95818815, 0.94773519, 0.95818815,
0.94076655, 0.94773519, 0.93728223, 0.95121951, 0.92682927,
0.93728223, 0.91986063, 0.92334495, 0.91289199, 0.92334495,
0.9825784 , 0.9825784 , 0.96515679, 0.9825784 , 0.97212544,
0.97560976, 0.95121951, 0.97560976, 0.94425087, 0.96515679,
0.94773519, 0.96515679, 0.94425087, 0.95121951, 0.94425087,
0.94425087, 0.94425087, 0.94425087, 0.94425087, 0.94425087]),
'split3_test_score': array([0.96515679, 0.96515679, 0.95470383, 0.96515679, 0.94773519,
0.94773519, 0.95470383, 0.95818815, 0.95470383, 0.95470383,
0.95470383, 0.95121951, 0.95470383, 0.95470383, 0.95121951,
0.95470383, 0.94425087, 0.95121951, 0.96864111, 0.95818815,
0.96167247, 0.96167247, 0.94076655, 0.96167247, 0.95818815,
0.95818815, 0.95121951, 0.96167247, 0.95470383, 0.95818815,
0.95818815, 0.95818815, 0.96515679, 0.96515679, 0.96167247,
0.96167247, 0.96515679, 0.96515679, 0.96167247, 0.96515679,
0.93728223, 0.93728223, 0.94425087, 0.93728223, 0.94773519,
0.94425087, 0.94773519, 0.95121951, 0.95818815, 0.95818815,
0.94773519, 0.95818815, 0.94773519, 0.95121951, 0.95121951,
0.95121951, 0.94773519, 0.95121951, 0.94425087, 0.95121951,
0.96515679, 0.96515679, 0.95470383, 0.96515679, 0.94773519,
0.94773519, 0.95470383, 0.95818815, 0.95470383, 0.95470383,
0.95470383, 0.95121951, 0.95470383, 0.95470383, 0.95121951,
0.95470383, 0.94425087, 0.95121951, 0.96864111, 0.95818815,
0.96515679, 0.96515679, 0.95470383, 0.96515679, 0.94773519,
0.94773519, 0.95470383, 0.95818815, 0.95470383, 0.95470383,
0.95470383, 0.95121951, 0.95470383, 0.95470383, 0.95121951,
0.95470383, 0.94425087, 0.95121951, 0.96864111, 0.95818815,
0.96167247, 0.96167247, 0.94076655, 0.96167247, 0.95818815,
0.95818815, 0.95470383, 0.96167247, 0.95470383, 0.95818815,
0.95818815, 0.95818815, 0.96864111, 0.96515679, 0.95818815,
0.95818815, 0.96515679, 0.96515679, 0.96167247, 0.96864111,
0.94076655, 0.94076655, 0.93379791, 0.93379791, 0.95818815,
0.95818815, 0.95470383, 0.95818815, 0.95470383, 0.95818815,
0.94425087, 0.95470383, 0.94076655, 0.94425087, 0.95818815,
0.95470383, 0.95121951, 0.95121951, 0.94425087, 0.95121951,
0.96515679, 0.96515679, 0.95470383, 0.96515679, 0.94773519,
0.94773519, 0.95470383, 0.95818815, 0.95470383, 0.95470383,
0.95470383, 0.95121951, 0.95470383, 0.95470383, 0.95121951,
0.95470383, 0.94425087, 0.95121951, 0.96864111, 0.95818815]),
'split4_test_score': array([0.98606272, 0.98606272, 0.9825784 , 0.98606272, 0.98954704,
0.98606272, 0.97560976, 0.9825784 , 0.9825784 , 0.97560976,
0.97909408, 0.97909408, 0.9825784 , 0.9825784 , 0.9825784 ,
0.98606272, 0.9825784 , 0.9825784 , 0.97909408, 0.9825784 ,
0.98606272, 0.98606272, 0.9825784 , 0.98606272, 0.9825784 ,
0.97909408, 0.97212544, 0.98606272, 0.97909408, 0.97909408,
0.97560976, 0.97909408, 0.97560976, 0.97909408, 0.97212544,
0.97909408, 0.97212544, 0.97212544, 0.97212544, 0.97909408,
0.96515679, 0.96515679, 0.95121951, 0.95121951, 0.96167247,
0.95818815, 0.95470383, 0.96167247, 0.96167247, 0.96515679,
0.96167247, 0.95818815, 0.96167247, 0.97212544, 0.96167247,
0.97212544, 0.96167247, 0.96515679, 0.95470383, 0.96167247,
0.98606272, 0.98606272, 0.9825784 , 0.98606272, 0.98954704,
0.98606272, 0.97560976, 0.9825784 , 0.9825784 , 0.97560976,
0.97909408, 0.97909408, 0.9825784 , 0.9825784 , 0.9825784 ,
0.98606272, 0.9825784 , 0.9825784 , 0.97909408, 0.9825784 ,
0.98606272, 0.98606272, 0.9825784 , 0.98606272, 0.98954704,
0.98606272, 0.97560976, 0.9825784 , 0.9825784 , 0.97560976,
0.97909408, 0.97909408, 0.9825784 , 0.9825784 , 0.9825784 ,
0.98606272, 0.9825784 , 0.9825784 , 0.97909408, 0.9825784 ,
0.9825784 , 0.9825784 , 0.9825784 , 0.98606272, 0.97909408,
0.97909408, 0.97212544, 0.98606272, 0.97560976, 0.97909408,
0.97560976, 0.97909408, 0.97560976, 0.97909408, 0.97212544,
0.97909408, 0.97212544, 0.97212544, 0.96864111, 0.97909408,
0.96864111, 0.96864111, 0.95121951, 0.95470383, 0.96515679,
0.96167247, 0.95818815, 0.96515679, 0.96864111, 0.96864111,
0.96167247, 0.96864111, 0.96515679, 0.97212544, 0.96515679,
0.97560976, 0.96864111, 0.96864111, 0.96515679, 0.96864111,
0.98606272, 0.98606272, 0.9825784 , 0.98606272, 0.98954704,
0.98606272, 0.97560976, 0.9825784 , 0.9825784 , 0.97560976,
0.97909408, 0.97909408, 0.9825784 , 0.9825784 , 0.9825784 ,
0.98606272, 0.9825784 , 0.9825784 , 0.97909408, 0.9825784 ]),
'mean_test_score': array([0.97287069, 0.97287069, 0.96590447, 0.97287069, 0.96868709,
0.96868709, 0.95755662, 0.96938637, 0.95894551, 0.96242741,
0.95686218, 0.9638163 , 0.95686218, 0.95895035, 0.95338753,
0.95825348, 0.95407714, 0.95616531, 0.95686943, 0.95617015,
0.96312911, 0.96312911, 0.95200106, 0.96382356, 0.96590689,
0.96660376, 0.94990563, 0.96591415, 0.95199139, 0.95825348,
0.94851916, 0.95616531, 0.94852158, 0.95130178, 0.9478223 ,
0.95199623, 0.94991047, 0.95060492, 0.94921603, 0.95200106,
0.95129694, 0.95129694, 0.94155536, 0.94850707, 0.94781746,
0.9512921 , 0.94990321, 0.95199381, 0.94990805, 0.95547329,
0.94850949, 0.95129694, 0.94225223, 0.94991047, 0.94086334,
0.94503968, 0.94015921, 0.94294425, 0.93946235, 0.94503 ,
0.97287069, 0.97287069, 0.96590447, 0.97287069, 0.96868709,
0.96868709, 0.95755662, 0.96938637, 0.95894551, 0.96242741,
0.95686218, 0.9638163 , 0.95686218, 0.95895035, 0.95338753,
0.95825348, 0.95407714, 0.95616531, 0.95686943, 0.95617015,
0.97287069, 0.97287069, 0.96590447, 0.97287069, 0.96868709,
0.96868709, 0.95755662, 0.96938637, 0.95894551, 0.96242741,
0.95686218, 0.9638163 , 0.95686218, 0.95895035, 0.95338753,
0.95825348, 0.95407714, 0.95616531, 0.95686943, 0.95617015,
0.96243225, 0.96243225, 0.95199864, 0.96382356, 0.96521003,
0.96660376, 0.9506025 , 0.96591415, 0.95198897, 0.95894793,
0.94921361, 0.95616531, 0.94921845, 0.95130178, 0.94712544,
0.95129936, 0.95060492, 0.95129936, 0.94782472, 0.95269793,
0.95685734, 0.95685734, 0.94293457, 0.94919909, 0.95476432,
0.95754694, 0.95338269, 0.95616773, 0.95060492, 0.95547571,
0.94503242, 0.95060492, 0.94433556, 0.9506025 , 0.94642373,
0.95199139, 0.94294425, 0.94780778, 0.94293215, 0.94711334,
0.97287069, 0.97287069, 0.96590447, 0.97287069, 0.96868709,
0.96868709, 0.95755662, 0.96938637, 0.95894551, 0.96242741,
0.95686218, 0.9638163 , 0.95686218, 0.95895035, 0.95338753,
0.95825348, 0.95407714, 0.95616531, 0.95686943, 0.95617015]),
'std_test_score': array([0.01446302, 0.01446302, 0.01882305, 0.01446302, 0.01954636,
0.01904299, 0.01692484, 0.01762635, 0.01750928, 0.01511592,
0.0143383 , 0.01417596, 0.01724968, 0.01693139, 0.02136241,
0.02026519, 0.01883609, 0.01885603, 0.02019405, 0.01922411,
0.02298839, 0.02298839, 0.02683849, 0.02371317, 0.01957004,
0.01921757, 0.0220214 , 0.02358461, 0.02234629, 0.02355308,
0.02379142, 0.02351891, 0.02459221, 0.02672602, 0.02385512,
0.02318607, 0.02267064, 0.02317823, 0.02297895, 0.02368955,
0.01756458, 0.01756458, 0.01476016, 0.01107147, 0.01519043,
0.01241204, 0.01231908, 0.01303417, 0.010848 , 0.01284938,
0.01286752, 0.01258343, 0.01289171, 0.01559561, 0.01581057,
0.01647874, 0.01497725, 0.01667988, 0.0136416 , 0.01528138,
0.01446302, 0.01446302, 0.01882305, 0.01446302, 0.01954636,
0.01904299, 0.01692484, 0.01762635, 0.01750928, 0.01511592,
0.0143383 , 0.01417596, 0.01724968, 0.01693139, 0.02136241,
0.02026519, 0.01883609, 0.01885603, 0.02019405, 0.01922411,
0.01446302, 0.01446302, 0.01882305, 0.01446302, 0.01954636,
0.01904299, 0.01692484, 0.01762635, 0.01750928, 0.01511592,
0.0143383 , 0.01417596, 0.01724968, 0.01693139, 0.02136241,
0.02026519, 0.01883609, 0.01885603, 0.02019405, 0.01922411,
0.02232589, 0.02232589, 0.02555343, 0.02371317, 0.01901824,
0.01921757, 0.02210692, 0.02358461, 0.02054709, 0.02244415,
0.02431536, 0.02351891, 0.0250979 , 0.02672602, 0.02348842,
0.02293578, 0.02156137, 0.02207262, 0.0235342 , 0.02411374,
0.01311811, 0.01311811, 0.01434876, 0.01293827, 0.01206191,
0.00744489, 0.01192058, 0.01150772, 0.01124606, 0.0114562 ,
0.01304742, 0.01357734, 0.01298769, 0.01494575, 0.01434685,
0.01414058, 0.01847563, 0.01691109, 0.0171665 , 0.01547699,
0.01446302, 0.01446302, 0.01882305, 0.01446302, 0.01954636,
0.01904299, 0.01692484, 0.01762635, 0.01750928, 0.01511592,
0.0143383 , 0.01417596, 0.01724968, 0.01693139, 0.02136241,
0.02026519, 0.01883609, 0.01885603, 0.02019405, 0.01922411]),
'rank_test_score': array([ 1, 1, 30, 1, 17, 17, 63, 13, 54, 45, 72, 37, 72,
49, 100, 58, 96, 87, 68, 82, 41, 41, 106, 35, 29, 25,
131, 27, 111, 58, 138, 87, 137, 114, 142, 109, 129, 123, 134,
107, 118, 118, 157, 140, 143, 121, 132, 110, 130, 94, 139, 118,
156, 128, 158, 148, 159, 152, 160, 150, 1, 1, 30, 1, 17,
17, 63, 13, 54, 45, 72, 37, 72, 49, 100, 58, 96, 87,
68, 82, 1, 1, 30, 1, 17, 17, 63, 13, 54, 45, 72,
37, 72, 49, 100, 58, 96, 87, 68, 82, 43, 43, 108, 35,
34, 25, 126, 27, 113, 53, 135, 87, 133, 114, 145, 116, 123,
116, 141, 105, 80, 80, 154, 136, 95, 67, 104, 86, 123, 93,
149, 122, 151, 126, 147, 111, 152, 144, 155, 146, 1, 1, 30,
1, 17, 17, 63, 13, 54, 45, 72, 37, 72, 49, 100, 58,
96, 87, 68, 82], dtype=int32)}
search.best_params_
{'algorithm': 'ball_tree',
'metric': 'euclidean',
'n_neighbors': 1,
'weights': 'uniform'}
2. Random Search Cross Validation.#
It performs the search in the brute-force way using cross-validation. One has to define the parameter space. The scikit-learn function is GridSearchCV
. More details here.
The advantage is that it can be used for a wide hyperparameter space and limit to n_iter
number of iterations.
from sklearn.model_selection import RandomizedSearchCV
from scipy.stats import randint
distributions= [ {'n_neighbors': randint.rvs(low=1,high=10,size=10), 'weights': ['uniform','distance'], 'algorithm': [ 'ball_tree', 'kd_tree'],
'metric':['euclidean','manhattan','chebyshev','minkowski']} ]
clf2 = RandomizedSearchCV(clf, distributions, random_state=0,cv=5,n_iter=100)
clf2.fit(X_train,y_train)
RandomizedSearchCV(cv=5, estimator=KNeighborsClassifier(n_neighbors=3), n_iter=100, param_distributions=[{'algorithm': ['ball_tree', 'kd_tree'], 'metric': ['euclidean', 'manhattan', 'chebyshev', 'minkowski'], 'n_neighbors': array([5, 4, 2, 8, 2, 6, 1, 1, 3, 5]), 'weights': ['uniform', 'distance']}], random_state=0)In a Jupyter environment, please rerun this cell to show the HTML representation or trust the notebook.
On GitHub, the HTML representation is unable to render, please try loading this page with nbviewer.org.
RandomizedSearchCV(cv=5, estimator=KNeighborsClassifier(n_neighbors=3), n_iter=100, param_distributions=[{'algorithm': ['ball_tree', 'kd_tree'], 'metric': ['euclidean', 'manhattan', 'chebyshev', 'minkowski'], 'n_neighbors': array([5, 4, 2, 8, 2, 6, 1, 1, 3, 5]), 'weights': ['uniform', 'distance']}], random_state=0)
KNeighborsClassifier(n_neighbors=3)
KNeighborsClassifier(n_neighbors=3)
print(search.best_params_)
print(clf2.best_params_)
{'algorithm': 'ball_tree', 'metric': 'euclidean', 'n_neighbors': 1, 'weights': 'uniform'}
{'weights': 'distance', 'n_neighbors': 2, 'metric': 'euclidean', 'algorithm': 'kd_tree'}